22-1-合同上传(代码)
大约 2 分钟
22-1-合同上传(代码)
1.需求说明
- 确定前端位置对合同进行上传
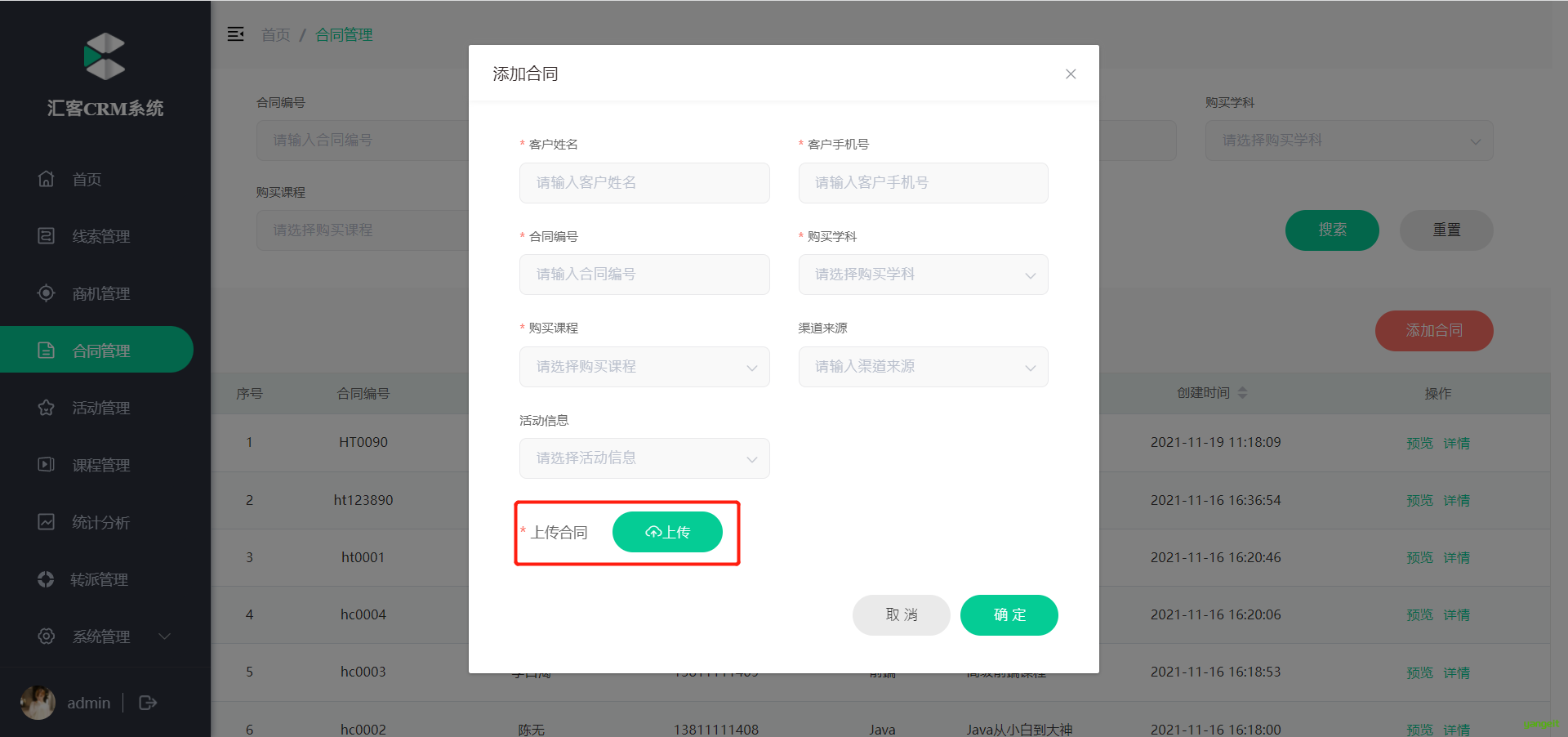
- 基于接口文档创建接口
合同上传
- 接口名:
/common/upload
- 请求方式:
POST
- 传入参数:
- 上传的文件
- 返回值:
{
"msg":"操作成功",
// 文件的位置和名字
"fileName":"/huike-crm/upload/2022/03/18/8916214c-44ff-4aef-83bb-dbc7d3f88ed8.pdf",
"code":200,
// 文件的访问链接
"url":"http://127.0.0.1:9000/huike-crm/upload/2022/03/18/8916214c-44ff-4aef-83bb-dbc7d3f88ed8.pdf"
}
2.实现步骤
2.1准备环境
检查依赖导入
<dependency>
<groupId>io.minio</groupId>
<artifactId>minio</artifactId>
<version>8.3.7</version>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>4.9.0</version>
</dependency>
2.2 书写接口
CommonController
/**
* 通用上传请求
*/
@PostMapping("/common/upload")
public AjaxResult uploadFile(MultipartFile file) throws Exception{
try{
// 上传文件路径
return fileService.upload(file);
}catch (Exception e){
return AjaxResult.error(e.getMessage());
}
}
2.3 业务层
ISysFileService
/**
* 文件上传
* @param file
* @return
*/
AjaxResult upload(MultipartFile file);
SysFileService
/**
* 文件上传至Minio
*/
@Override
public AjaxResult upload(MultipartFile file) {
InputStream inputStream = null;
//1.创建Minio的连接对象
MinioClient minioClient = getClient();
//2.获得桶名字
String bucketName = minioConfig.getBucketName();
try {
inputStream = file.getInputStream();
//3.判断文件存储的桶是否存在
boolean found = minioClient.bucketExists(BucketExistsArgs.builder().bucket(bucketName).build());
if (!found) {
//4.如果桶不存在则创建通
minioClient.makeBucket(MakeBucketArgs.builder().bucket(bucketName).build());
}
//5.操作文件,取一个名字
String fileName = file.getOriginalFilename();
String objectName = new SimpleDateFormat("yyyy/MM/dd/").format(new Date()) + UUID.randomUUID().toString().replaceAll("-", "")
+ fileName.substring(fileName.lastIndexOf("."));
//6.文件上传
//由于使用的是SpringBoot与之进行集成 上传的时候拿到的是MultipartFile 需要通过输入输出流的方式进行添加
PutObjectArgs objectArgs = PutObjectArgs.builder().object(objectName)
.bucket(bucketName)
.contentType(file.getContentType())
.stream(file.getInputStream(),file.getSize(),-1).build();
minioClient.putObject(objectArgs);
//7.封装访问的url给前端
AjaxResult ajax = AjaxResult.success();
ajax.put("fileName", "/"+bucketName+"/"+objectName);
//url需要进行截取
ajax.put("url", minioConfig.getEndpoint()+":"+ minioConfig.getPort()+"/"+ minioConfig.getBucketName()+"/"+fileName);
return ajax;
}catch(Exception e){
e.printStackTrace();
return AjaxResult.error("上传失败");
}finally {
//防止内存泄漏
if (inputStream != null) {
try {
inputStream.close(); // 关闭流
} catch (IOException e) {
log.debug("inputStream close IOException:" + e.getMessage());
}
}
}
}
@Autowired
MinioConfig minioConfig;
/**
* 获取Minio连接
* @return
*/
private MinioClient getClient(){
MinioClient minioClient =
MinioClient.builder()
.endpoint("http://"+minioConfig.getEndpoint()+":"+ minioConfig.getPort())
.credentials(minioConfig.getAccessKey(),minioConfig.getSecretKey())
.build();
return minioClient;
}